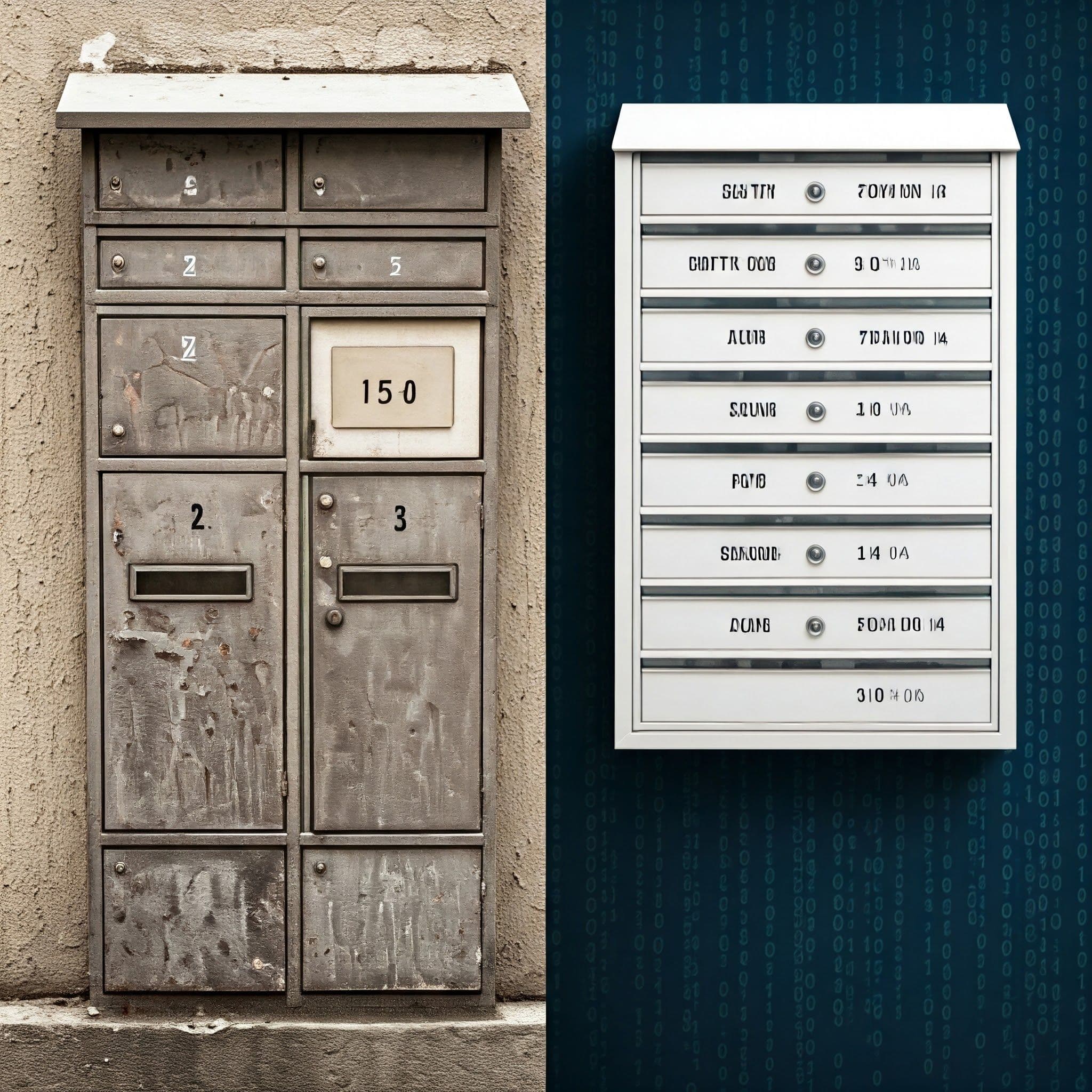
Self-Documenting TypeScript: Why Meaningful Generic Names Matter in 2025

Ohhh that good old days when saving a few keystrokes meant the difference between your code fitting on a 80x24 terminal screen or not, Doom, Quake, purtty and vim. Well, it's 2025, and if you're still using single-letter generics, you might also be coding on a punch card. Let's dive into why descriptive generic names are not just a luxury but a necessity in modern TypeScript development.
Historical Context: From T to Clarity
Back in 1973, when bell-bottoms were in fashion and the ML (Meta Language) programming language was born, using T
for "Type" made perfect sense. Storage was expensive, screens were tiny, and typing more characters actually took considerable effort on the keyboards of that era. The tradition stuck, giving us classics like:
T
for Type (because apparently, "Type" was too long to type)K
for Key (not to be confused with the actual keyboard key)V
for Value (no relation to "V for Vendetta")E
for Element (E.T. would be proud)R
for Return type (because "Return" was apparently too mainstream)
But just as we've moved on from 80x24 terminals to 4K display (or two), perhaps it's time to move on from single-letter generics too.
The Case for Descriptive Generics: Because Your Future Self Will Thank You
Example 1: API Response Handler - From Cryptic to Crystal Clear
Let's look at a common scenario: handling API responses. Consider this familiar pattern that you might see in many codebases:
// The "before" version - cryptic and mysterious
interface ApiResponse<T> {
data: T;
status: number;
timestamp: Date;
}
// How it's used - good luck remembering what T represents six months later 😁
const response: ApiResponse<UserData> = await fetchUser(id);
Now, let's make it self-documenting:
// The "after" version - crystal clear and self-documenting 🔥
interface ApiResponse<ResponseData> {
data: ResponseData;
status: number;
timestamp: Date;
}
// How it's used - immediately obvious what we're dealing with ❤️
const response: ApiResponse<UserData> = await fetchUser(id);
In the real world, you might be dealing with multiple nested generic parameters. Which version would you rather debug at 3 AM during a production incident?
Example 2: State Management - Because State is Complicated Enough Already
State management is tricky enough without adding cryptic type parameters to the mix. Here's how we can make it more readable:
// Before: What do S and A stand for? Your guess is as good as mine
class StateManager<S, A> {
private state: S;
dispatch(action: A): void {
// Implementation that you'll decipher someday, maybe
}
}
// After: Ah, now we're speaking human!
class StateManager<State, Action> {
private state: State;
dispatch(action: Action): void {
// Implementation that actually makes sense when you read it
}
}
The beauty of this approach is that when you come back to this code months later, you won't need to play detective to figure out what these types represent.
Example 3: Financial Operations - Because Money Deserves Respect
When dealing with financial operations, clarity isn't just nice to have – it's essential. Here's how we can make currency conversions more explicit:
// Before: A recipe for financial disaster
type Money<T> = {
amount: number;
currency: T;
}
function convert<T, U>(from: Money<T>, rate: number): Money<U> {
// Hope you remember what T and U mean when auditing this code
}
// After: Crystal clear and audit-friendly
type Money<Currency extends AllowedCurrencies> = {
amount: number;
currency: Currency;
}
function convert<FromCurrency extends AllowedCurrencies, ToCurrency extends AllowedCurrencies>(
from: Money<FromCurrency>,
exchangeRate: ExchangeRate<FromCurrency, ToCurrency>
): Money<ToCurrency> {
// Even your accountant could understand this
}
When you're dealing with financial calculations, the last thing you want is ambiguity. Explicit type names can help prevent costly mistakes and make code reviews much more effective.
Example 4: Collection Operations - Transform Your Understanding
Collection operations are common in any application. Let's make them more understandable:
// Before: Classic but cryptic
function transform<T, U>(items: T[], fn: (item: T) => U): U[] {
return items.map(fn);
}
// After: Self-documenting and clear
function transform<InputItem, TransformedItem>(
items: InputItem[],
transformer: (item: InputItem) => TransformedItem
): TransformedItem[] {
return items.map(transformer);
}
// Usage becomes more intuitive:
const numbers = [1, 2, 3];
const strings = transform<number, string>(
numbers,
num => num.toString()
);
Benefits of Descriptive Generic Names: The Gift That Keeps on Giving
- Self-Documentation: Your code tells a story that anyone can understand. No more mysterious single letters that make your codebase look like a spy's notebook.
- Improved Code Review: Your reviewers won't need to play Sherlock Holmes to understand your generic parameters. They can focus on what your code does rather than what your types mean.
- Better IDE Support: Modern IDEs are smart, but they can't read minds. Descriptive names make autocomplete suggestions actually meaningful. No more "Did I want T1 or T2 here?"
- Reduced Cognitive Load: Your brain has better things to do than maintaining a mental mapping of single letters to their meanings. Save that mental energy for solving actual problems.
- Enhanced Maintainability: Future maintainers (including future you) will be able to understand your code without needing to read through layers of implementation details or documentation.
When to Use Single-Letter Generics: Yes, They Still Have Their Place
While we're advocating for more descriptive names, there are still legitimate uses for single-letter generics. Here's when they make sense:
Simple Utility Types
When the context is so clear that adding more characters would just be noise:
// These are fine - the usage is obvious and universal
type Nullable<T> = T | null;
type Optional<T> = T | undefined;
Standard Library Patterns
When following established patterns that everyone knows:
// Following conventions from Array.map, Promise.all, etc.
array.map<T>(mapper: (item: T) => U): U[]
Real-World Impact: A Case Study
Let's look at a real-world scenario. Imagine you're maintaining a large e-commerce application:
// Before: Good luck understanding this six months later
interface CatalogQuery<T, F, S> {
filters: F;
sort: S;
transform: (item: T) => T;
}
// After: Immediately clear what each parameter represents
interface CatalogQuery<ItemType, FilterCriteria, SortOptions> {
filters: FilterCriteria;
sort: SortOptions;
transform: (item: ItemType) => ItemType;
}
In an age where we have infinite scroll on 4K monitors and IDEs that can practically read our minds, saving a few characters in our type names is a false economy. The small effort of typing out descriptive generic names pays massive dividends in code readability and maintainability.
Remember: code is written once but read many times. Your future self, your team members, and anyone else who maintains your code will thank you for taking the time to write clear, self-documenting generic names.
So the next time you're tempted to use T
, ask yourself: "Will someone reading this code understand what this type parameter represents without needing to dive into the implementation?" If the answer is no, take a few extra keystrokes and make it clear. Your future self will thank you for it.
And hey, if you're still using single-letter generics in 2025, maybe it's time to also reconsider that Comic Sans font in your IDE?
P.S. Yes, you can use Comic Neue in your IDE, it's 2025 now 😁